In this article, I will explain 'How We Dynamically Generate Word Document Report in ASP.NET with HTML'. For generate report we can uses many third party DLLs in asp.net but we can easily generate Ms Word document reports using HTML content.
Here I will create a runtime HTML document using StringBuilder class which is very efficient for string manipulation. After that render the response as 'application/msword' mime type.
The following example shown how to place heading and table dynamically in the word document in ASP.NET or VB.NET using HTML.
.aspx Page Code:
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Runtime Generating MS Word Document Report in ASP.NET C# VB.NET with HTML (www.webcodelogics.com)</title>
<style>
table, td, th {
border: 1pt solid #1FB5AD;
font-family: arial;
}
td, th {
padding: 2px 5px;
}
</style>
</head>
<body style="background: #E0EDFA; text-align: center; margin-top: 250px; font-family: Arial;">
<form id="form1" runat="server">
<div>
<h2>Generate Word File</h2>
<asp:Button ID="btnGenerateDocReport" runat="server" OnClick="btnGenerateDocReport_Click" ToolTip="Generate Word File" Text="Generate Word File"></asp:Button>
<br />
<br />
<table cellspacing='0' cellpadding='0' width="200px" style="margin: auto;">
<tr>
<th style='background: #1FB5AD; color: #fff;'>S.No.</th>
<th style='background: #1FB5AD; color: #fff;'>Name</th>
</tr>
<tr>
<td>1.</td>
<td>Harry</td>
</tr>
<tr>
<td>2.</td>
<td>John</td>
</tr>
<tr>
<td>3.</td>
<td>Martin</td>
</tr>
</table>
</div>
</form>
</body>
</html>
<head runat="server">
<title>Runtime Generating MS Word Document Report in ASP.NET C# VB.NET with HTML (www.webcodelogics.com)</title>
<style>
table, td, th {
border: 1pt solid #1FB5AD;
font-family: arial;
}
td, th {
padding: 2px 5px;
}
</style>
</head>
<body style="background: #E0EDFA; text-align: center; margin-top: 250px; font-family: Arial;">
<form id="form1" runat="server">
<div>
<h2>Generate Word File</h2>
<asp:Button ID="btnGenerateDocReport" runat="server" OnClick="btnGenerateDocReport_Click" ToolTip="Generate Word File" Text="Generate Word File"></asp:Button>
<br />
<br />
<table cellspacing='0' cellpadding='0' width="200px" style="margin: auto;">
<tr>
<th style='background: #1FB5AD; color: #fff;'>S.No.</th>
<th style='background: #1FB5AD; color: #fff;'>Name</th>
</tr>
<tr>
<td>1.</td>
<td>Harry</td>
</tr>
<tr>
<td>2.</td>
<td>John</td>
</tr>
<tr>
<td>3.</td>
<td>Martin</td>
</tr>
</table>
</div>
</form>
</body>
</html>
C# Code:
// Add the following namespaces.
using System;
using System.Text;
using System.Web;
// Add the following code in the button click event.
protected void btnGenerateDocReport_Click(object sender, EventArgs e)
{
StringBuilder strHtml = new StringBuilder();
strHtml.Append("<!DOCTYPE html PUBLIC '-//W3C//DTD XHTML 1.0 Transitional//EN http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd'>");strHtml.Append("<html xmlns='http://www.w3.org/1999/xhtml'>");
strHtml.Append("<head><META HTTP-EQUIV='Content-Type' CONTENT='text/html; charset=UTF-8'>");
strHtml.Append("<meta name=ProgId content=Word.Document>");
strHtml.Append("<meta name=Generator content='Microsoft Word 9'>");
strHtml.Append("<meta name=Originator content='Microsoft Word 9'>");
strHtml.Append("<title>demoWordFile(webcodelogics.com)</title><style>");
strHtml.Append("@page Section1 {size:595.45pt 841.7pt; margin:0.5in 0.5in 0.5in 0.5in;mso-header-margin:.5in;mso-footer-margin:.5in;mso-paper-source:0;}");
strHtml.Append("div.Section1 {page:Section1;}");
strHtml.Append("@page Section2 {size:841.7pt 595.45pt;mso-page-orientation:landscape;margin:0.5in 0.5in 0.5in 0.5in;mso-header-margin:.5in;mso-footer-margin:.5in;mso-paper-source:0;}");
strHtml.Append("div.Section2 {page:Section2;}");
strHtml.Append("</style></head><body><div class=Section2>");
strHtml.Append(" <table cellspacing='0' cellpadding='0' width='200' border='0' style='font-family: Open Sans,sans-serif;text-align:center;'> ");
strHtml.Append(" <tr><th style='background:#1FB5AD;color:#fff;border:1pt solid #1FB5AD;'>S.No.</th><th style='background:#1FB5AD;color:#fff;border:1pt solid #1FB5AD;'>Name</th></tr> ");
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>1.</td><td style='border:1pt solid #1FB5AD;'>Harry</td></tr> ");
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>2.</td><td style='border:1pt solid #1FB5AD;'>John</td></tr> ");
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>3.</td><td style='border:1pt solid #1FB5AD;'>Martin</td></tr> ");
strHtml.Append(" </table></div></body></html> ");
HttpContext.Current.Response.Clear();
HttpContext.Current.Response.Charset = "";
HttpContext.Current.Response.ContentType = "application/msword";
string strFileName = "demoWordFile(webcodelogics.com)" + ".doc";
HttpContext.Current.Response.AddHeader("Content-Disposition", "inline; filename=" + strFileName);
HttpContext.Current.Response.Write(strHtml);
HttpContext.Current.Response.End();
HttpContext.Current.Response.Flush();
}
VB.Net Code:
// Add the following code in the button click event.
Protected Sub btnGenerateDocReport_Click(sender As Object, e As EventArgs)
Dim strHtml As New StringBuilder()
strHtml.Append("<html xmlns='http://www.w3.org/1999/xhtml'>")
strHtml.Append("<head><META HTTP-EQUIV='Content-Type' CONTENT='text/html; charset=UTF-8'>")
strHtml.Append("<meta name=ProgId content=Word.Document>")
strHtml.Append("<meta name=Generator content='Microsoft Word 9'>")
strHtml.Append("<meta name=Originator content='Microsoft Word 9'>")
strHtml.Append("<title>demoWordFile(webcodelogics.com)</title><style>")
strHtml.Append("@page Section1 {size:595.45pt 841.7pt; margin:0.5in 0.5in 0.5in 0.5in;mso-header-margin:.5in;mso-footer-margin:.5in;mso-paper-source:0;}")
strHtml.Append("div.Section1 {page:Section1;}")
strHtml.Append("@page Section2 {size:841.7pt 595.45pt;mso-page-orientation:landscape;margin:0.5in 0.5in 0.5in 0.5in;mso-header-margin:.5in;mso-footer-margin:.5in;mso-paper-source:0;}")
strHtml.Append("div.Section2 {page:Section2;}")
strHtml.Append("</style></head><body><div class=Section2>")
strHtml.Append(" <table cellspacing='0' cellpadding='0' width='200' border='0' style='font-family: Open Sans,sans-serif;text-align:center;'> ")
strHtml.Append(" <tr><th style='background:#1FB5AD;color:#fff;border:1pt solid #1FB5AD;'>S.No.</th><th style='background:#1FB5AD;color:#fff;border:1pt solid #1FB5AD;'>Name</th></tr> ")
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>1.</td><td style='border:1pt solid #1FB5AD;'>Harry</td></tr> ")
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>2.</td><td style='border:1pt solid #1FB5AD;'>John</td></tr> ")
strHtml.Append(" <tr><td style='border:1pt solid #1FB5AD;'>3.</td><td style='border:1pt solid #1FB5AD;'>Martin</td></tr> ")
strHtml.Append(" </table></div></body></html> ")
Dim fileName As String = "demoWordFile(webcodelogics.com)" + ".doc"
Response.AppendHeader("Content-Type", "application/msword")
Response.AppendHeader("Content-Disposition", "attachment; filename=" & fileName)
Response.Write(strHtml.ToString())
End Sub
First clear the Response object, set charset to empty and set Content-Type to application/msword.
Define file name as per your choice for document generate through this above code and set this to "Content-Disposition" header of the Response object.
Set StringBuilder variable to Response object’s write.
Now, save the document and run it to your browser.
Click the 'Generate Word File' button in the screen, Then prompt will be displayed to open or save the document.
Result:
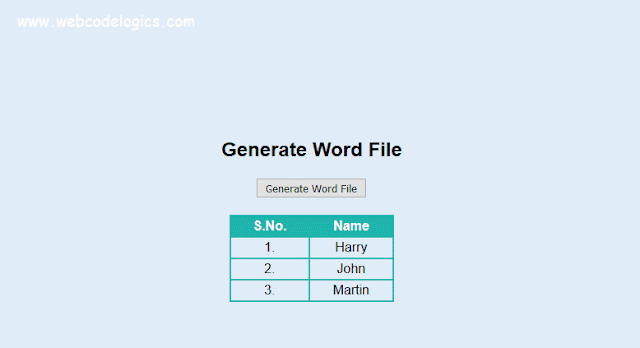
If you like this, please share with your friends...