Introduction:
In this article, we will learn about How to blink or flashing any text or image using simple CSS3 animation effect with example. or How to use animation effect in CSS3 to blink or flashing any text or image.
The preview are shown as below-
To blink/flash text or image write the code as shown below-
HTML:
Now save the code and run on browser to view the blink/flash text animation effect.
Demo:
webcodelogics.com
HTML:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>How to Blink or Flashing Text or Image Using Simple CSS3 with Example | webcodelogics.com</title> <style> .blink { -webkit-animation-name: blinker; -webkit-animation-duration: 1s; -webkit-animation-timing-function: linear; -webkit-animation-iteration-count: infinite; -moz-animation-name: blinker; -moz-animation-duration: 1s; -moz-animation-timing-function: linear; -moz-animation-iteration-count: infinite; animation-name: blinker; animation-duration: 1s; animation-timing-function: linear; animation-iteration-count: infinite; } @-moz-keyframes blinker { 0% { opacity: 1.0; } 50% { opacity: 0.0; } 100% { opacity: 1.0; } } @-webkit-keyframes blinker { 0% { opacity: 1.0; } 50% { opacity: 0.0; } 100% { opacity: 1.0; } } @keyframes blinker { 0% { opacity: 1.0; } 50% { opacity: 0.0; } 100% { opacity: 1.0; } } .block { text-align: center; width: 100%; } .block span{ font-size:25px; font-family:Arial; } .block img{ vertical-align: middle; margin-right:25px; } </style> </head> <body style="background: #E0EDFA;"> <div class="block"> <img src="http://webcodelogics.com/Images/logo.png" class="blink" alt="webcodelogics.com" /> <br /> <span class="blink">webcodelogics.com</span> </div> </body> </html>
Now save the code and run on browser to view the blink/flash text animation effect.
Demo:
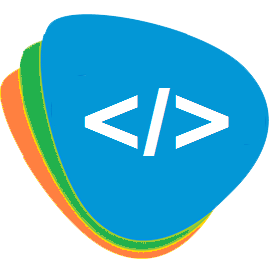